Introduction
Niva is strongly inspired by the forgotten Smalltalk language.
How learning Smalltalk can improve your skills as a programmer
Smalltalk tried to minimize the number of parentheses in it's syntax while remaining very similar to Lisp in its nature.
Instead of everything being a S-expression, everything is a message send.
So we can say that niva is a typed Lisp with much less parentheses, + methods and tagged unions.
On an imaginary graph of complexity, I would put it here:
Go < Niva < Java < Kotlin < Scala
Niva is my experiment in creating a typed Smalltalk with some crazy "what if" features.
Here some bullet points:
Minimalistic Smalltalk-like syntax with types
Tagged unions are the main way to achieve polymorphism instead of inheritance.
Prefer static to dynamism, the code is easier to understand because you always know which specific method will be called.
No method overloading for same receiver, no methods @override, no late binding
There are no functions, everything is method, in other words there is always a receiver,
"Hello" echo
instead ofecho("Hello")
, print is a message for String, not a top level functionHeavy lambda utilization. They are much easier to create(
[42]
is valid lambda that returns 42) and free to use thanks to inliningThere are no if\while\do while statements, everything is a message send, like in Smalltalk with a few exceptions for type declaration and matching
Null safety (Swift\Kotlin like nullable types)
Errors are between values and exceptions(Nim\Roc-like effects) and all possible errors of the scope is union that can be exhaustively matched
No imports. Nowadays modern IDEs are making imports for you, so why not move this feature to the language level. As long as the types do not completely match in name and field names, the imports will be inferred automatically
Application level language.
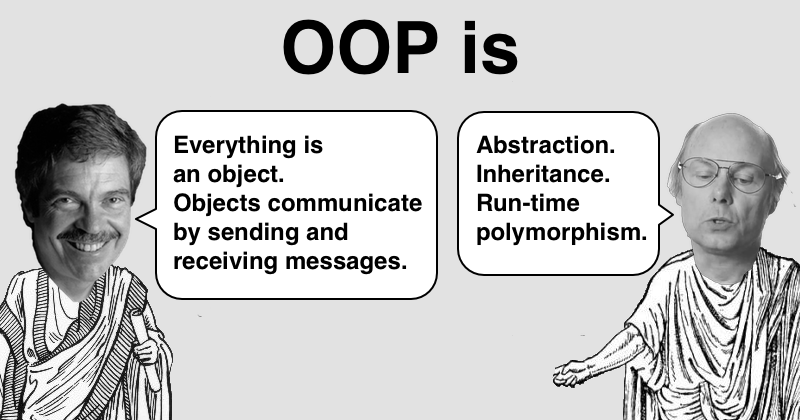
Syntax without much explanation
Here are syntax examples to give you a general impression. Everything will be explained in detail later.
Target
Right now Niva targets JVM, and provide easy way to call any Java\Kotlin code.
Im planning to add more compilation targets for faster comp-time in the future.